Case study: Stripe documentation feedback system
How Stripe collects user feedbacks about its documentation pages with a step by step guide to replicate it in your own documentation with Feelback
In this article we’re going to analyze the Stripe documentation feedback system, how it works and how to add a similar system on your website.
How Stripe documentation collects feedback
At the end of each article, the Stripe documentation asks the user to evaluate the page. If you scroll down to the bottom, the page displays a question:

When the user click either the Yes or the No nothing is sent yet. Instead, a detailed form with multiple choice appears.
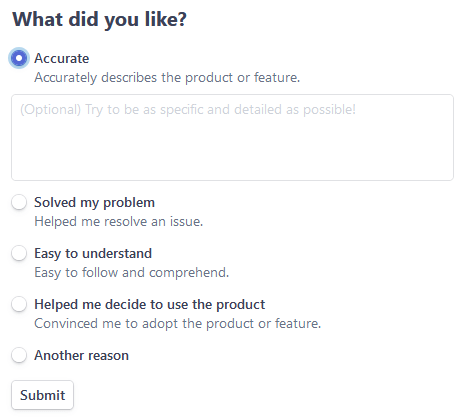
The form title and the choices are different for the Yes and the No feedback. In addition, for each choice, the user can type an optional message to further detail the feedback.
Let’s analyze in detail how the Stripe feedback collection works.
Where is asked?
Stripe documentation asks for feedback in all article pages. Some index, landing or marketing page doesn’t have a feedback form because, of course, it’s not the purpose of page. But, as a common convention, each documentation page includes the feedback question Was this page useful?.
Each documentation page provides valuable information to the user, so it’s useful to know what the user thinks about that information.
What is collected?
The Stripe documentation feedback is quite simple. It’s composed by two fields:
- a category (or label)
- a text message
To make the category selection as effortless as possible, the UI presents the categories as a set of predefined choices via radio buttons.
As last bit of emphasis, Stripe choose to have the message optional. This will further reduce the user psychological load and will result in an higher volume of feedback collected.
How is asked?
Stripe asks to evaluate the page by picking among predefined choices. To ease the process, the choices are broken down in two groups, with a simpler leading question.
The short question with a simple Yes/No choice is easily understood by the user, just with a glance. The common thumbs up / thumbs down buttons further help to communicate what is the expected interaction with familiar elements. In addition, the reveal mechanism reduces the user friction caused by a larger quantity of information to parse and digest. The less the info, the more likely the user will understand and act upon.
How to add a Stripe-like feedback system in your website
We’re going to use Feelback to easily add a Stripe-like feedback system on your website. In particular, we’ll use the Tagged Message feedback to collect categorized messages from your users.
Step 1: Identify feedback categories
The Stripe feedback system allows the user select a category for the feedback. To incorporate a similar system in your website, first of all, we need to identify all the different feedback categories the user can submit, and pick a unique name for each category.
In this guide, we’ll follow the same categories that Stripe uses.
For the positive feedback:
category | description |
---|---|
accurate | Accurate |
problem-solved | Helped solve my problem |
clear | Easy to understand |
product-chosen | Helped me decide to use the product |
yes-other | Another reason |
For the negative ones:
category | description |
---|---|
inaccurate | Inaccurate |
missing-info | Couldn’t find what I was looking for |
unclear | Hard to understand |
bad-examples | Code samples errors |
no-other | Another reason |
Step 2: Project setup
If you don't have already a Feelback account, please signup and create one. It's free and doesn't require a credit card to use.
Access the Feelback panel, and create a project if you don't have any.
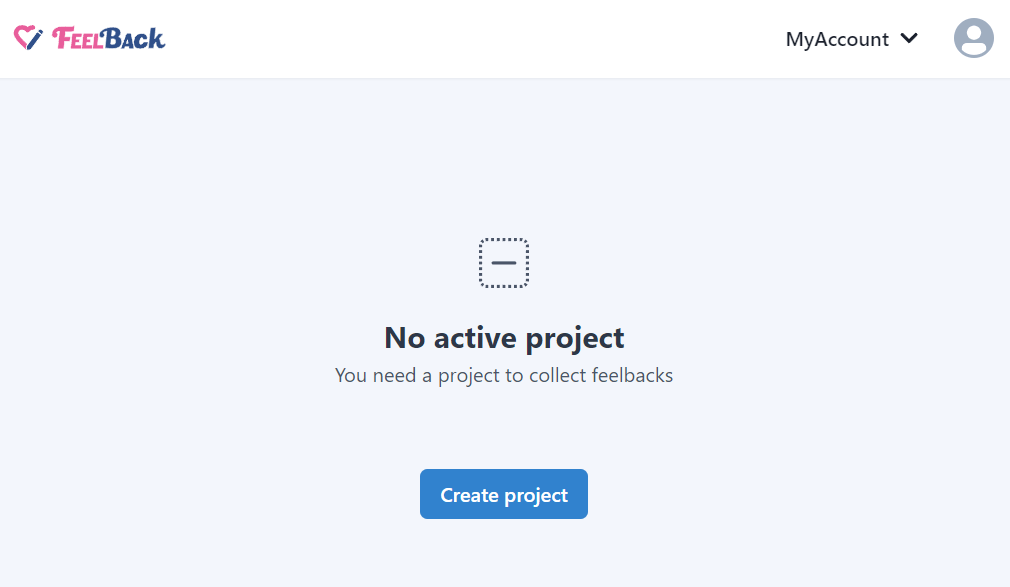
You can use your website name.
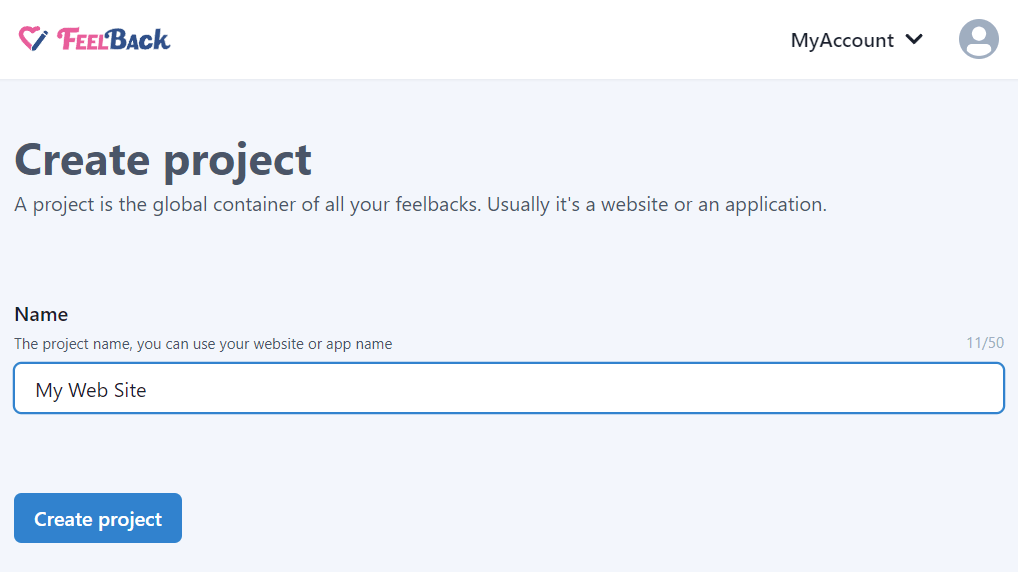
After that, create a ContentSet which will contain your content and feedbacks you will receive.
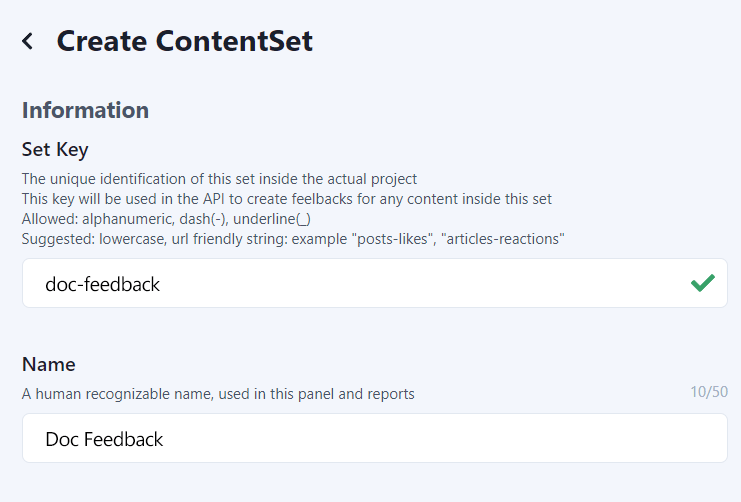
Pick the Tagged Message type to enable this content-set to receive categorized feedback message signals.
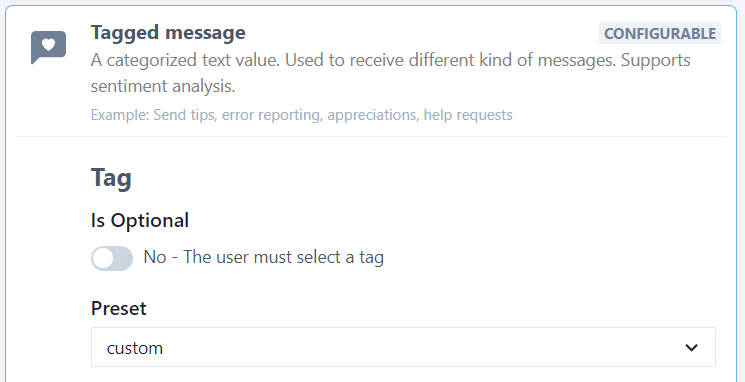
For the Tag, pick the custom preset which allows you to set custom values to model your feedbacks categories.
Turn on sentiment analysis to associate a positive or negative value to each category.

Now, you can insert the values and the relative sentiment:
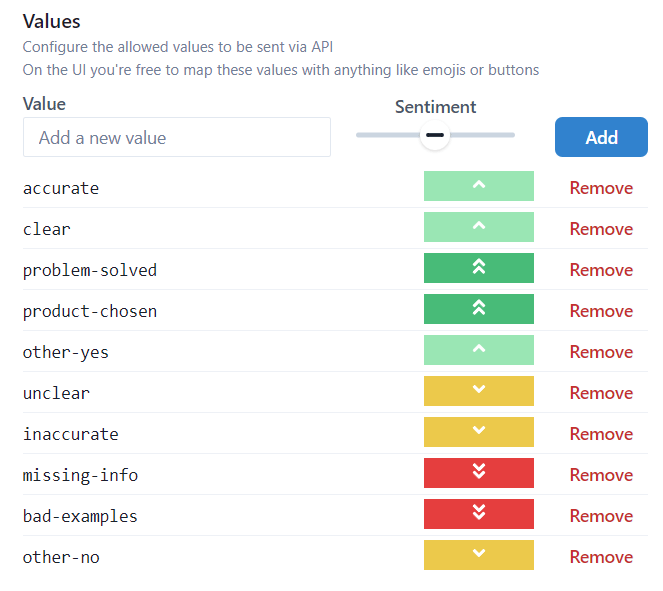
Set the Message optional, so the user not required to fill additional details.
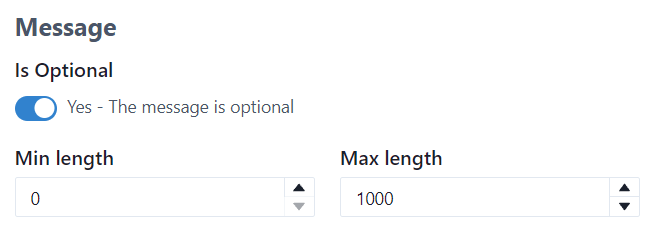
You will end up with a ContentSet like the following one:
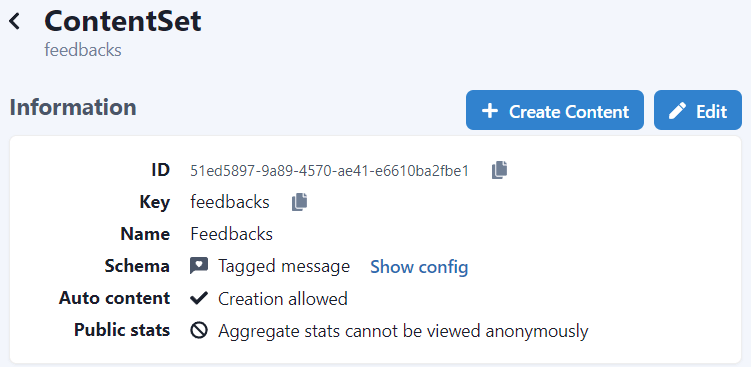
Step 3: Install the Feelback plugin
For this guide we’re going to assume you have a React-based website. Therefore, the next steps will focus on how to integrate a Feelback React component.
If your website is not build with React, Feelback offers integrations with many different libraries, static generators and site builders, where the same steps outlined here will apply.
For React, Feelback provides the dedicated @feelback/react
integration package. In your project directory, you can install it with your preferred package manager:
npm install @feelback/react
pnpm add @feelback/react
yarn add @feelback/react
Step 4: Create the Feelback component
Let’s start creating a dedicated component to encapsulate all feedback logic. Later, we can import the component in the page layout.
export function FeedbackComponent() {
return (
<div className="feelback-container">
</div>
);
}
The @feelback/react
package offers many builtin component and presets which helps to build your own feedback UX. You can check the React integration guide for extensive details about all provided components and presets.
For this case, we’ll use the Question
component to display the question to the user. Just import it together with the LIKE_DISLIKE
preset to show the buttons. Also, let’s include the predefined styling provided by the package.
import { Question, PRESET_YESNO_LIKE_DISLIKE } from "@feelback/react";
import "@feelback/react/styles/feelback.css";
export function FeedbackComponent() {
return (
<div className="feelback-container">
<Question text="Was this page helpful?"
items={PRESET_YESNO_LIKE_DISLIKE}
showLabels
/>
</div>
);
}
Now we can define the different Tag values, that is, the categories we have identified in first step
Let’s use the FeelbackValueDefinition
type to have nice editor autocompletion.
import { FeelbackValueDefinition, Question, PRESET_YESNO_LIKE_DISLIKE } from "@feelback/react";
const YES_TAGS: FeelbackValueDefinition[] = [
{ value: "accurate", title: "Accurate", description: "Accurately describes the product or feature.", },
{ value: "problem-solved", title: "Solved my problem", description: "Helped me resolve an issue.", },
{ value: "clear", title: "Easy to understand", description: "Easy to follow and comprehend.", },
{ value: "product-chosen", title: "Helped me decide to use the product", description: "Convinced me to adopt the product or feature.", },
{ value: "other-yes", title: "Another reason" },
];
const NO_TAGS: FeelbackValueDefinition[] = [
{ value: "inaccurate", title: "Inaccurate", description: "Doesn't accurately describe the product or feature.", },
{ value: "missing-info", title: "Couldn't find what I was looking for", description: "Missing important information.", },
{ value: "unclear", title: "Hard to understand", description: "Too complicated or unclear.", },
{ value: "bad-examples", title: "Code samples errors", description: "One or more code samples are incorrect.", },
{ value: "other-no", title: "Another reason" },
];
export function FeedbackComponent() {
/** omitted */
}
Finally, we’re introducing the main FeelbackTaggedMessage
component responsible to send the actual feedback.
Let’s display the various options when the user presses either the Yes or No button. Then, we can show the relative feedback options.
Here’s the final FeedbackComponent
component, which includes the predefined styling provided by the package.
import { useState } from "react";
import { FeelbackTaggedMessage, FeelbackValueDefinition, Question, PRESET_YESNO_LIKE_DISLIKE } from "@feelback/react";
import "@feelback/react/styles/feelback.css";
const YES_TAGS: FeelbackValueDefinition[] = [ /* omitted */ ];
const NO_TAGS: FeelbackValueDefinition[] = [ /* omitted */ ];
const FEEDBACK_CONTENT_SET_ID = "content-set-id-from-the-panel";
export function FeedbackComponent() {
const [choice, setChoice] = useState();
return (
<div className="feelback-container">
{!choice
? <Question text="Was this page helpful?"
items={PRESET_YESNO_LIKE_DISLIKE}
showLabels
onClick={setChoice}
/>
: <FeelbackTaggedMessage contentSetId={FEEDBACK_CONTENT_SET_ID}
layout="radio-group"
tags={choice === "y" ? YES_TAGS : NO_TAGS}
title={choice === "y" ? "What did you like?" : "What went wrong?"}
placeholder="(optional) Please, further detail the feedback"
/>
}
</div>
);
}
Step 5: Import the component
Now it’s time to import the newly created FeedbackComponent
inside your article page. Let’s suppose you have a Article
component for the documentation page.
Just import the component and add it at the end of the article content.
import { FeedbackComponent } from "./components/feedback.ts";
export function Article() {
return (
<article>
{/* article content here */}
<hr />
<FeelbackComponent />
</article>
);
}
Step 6: Customize the style (Optional)
You can override the predefined style with additional CSS.
For example, you can add some selector to change the buttons background.
custom.css
.feelback-container .feelback-btn {
background-color: "cyan";
}
Full documentation with advanced customization is available at the React integration guide.
Analyze aggregated feedback data
You can access the Feelback panel and checkout your content performance. A content item is the target object of the user feedback. In this scenario, a content item is the actual page the user is browsing.
In the dashboard, you can quickly see which page performs better.
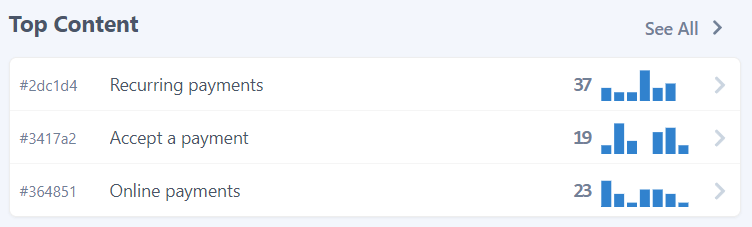
For each page you can analyze aggregated data, which include the feedback count received. You can go back and check historical data and change the aggregation period to week, month or year.
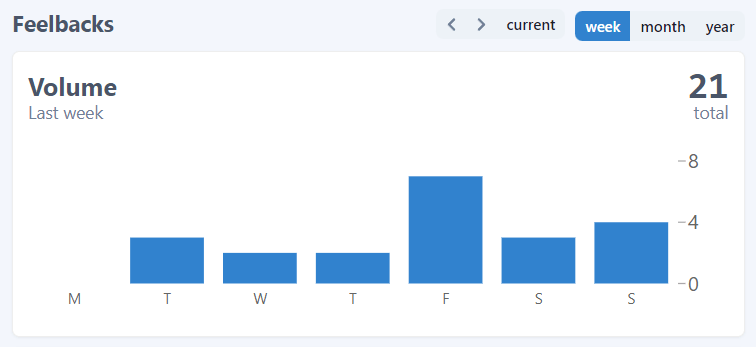
In this guide, we used the TaggedMessage to collect user feedbacks. And, because we configured sentiment values, Feelback creates sentiment aggregations too. For each page you can see the overall evaluation and the per Tag split.
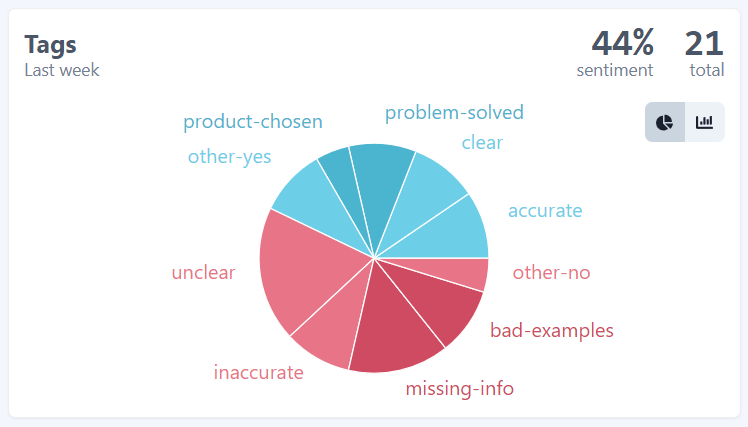
In addition to the aggregated view, you can go deep and analyze each single feedback. You can search, filter and check each feedback and see the details.
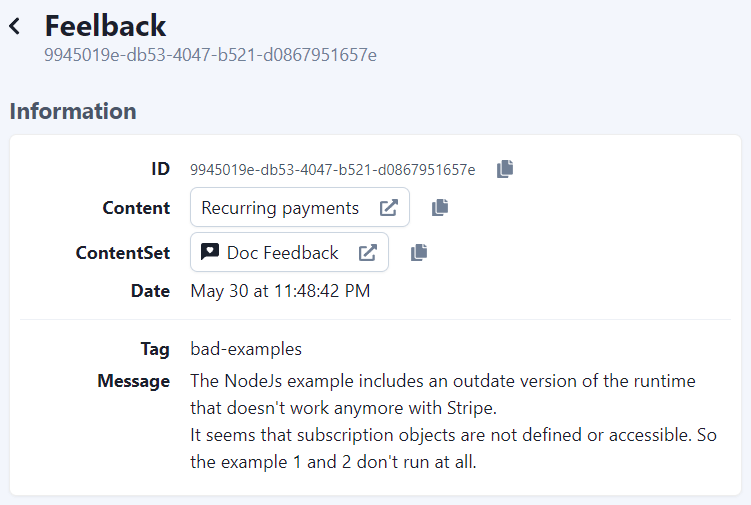
Each feedback comes with context data to further understand the relevance and specifics.
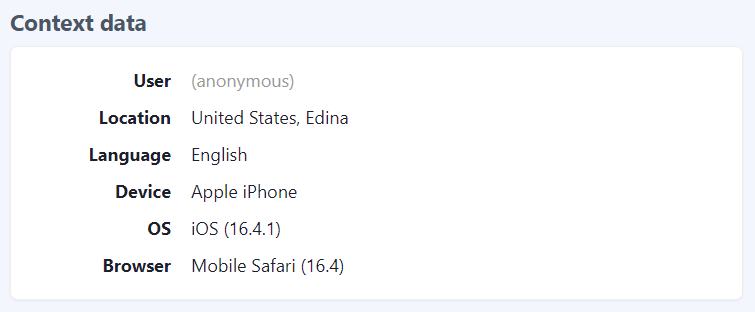
Additional resources
- Read the Feelback types list to know all signals you can receive from your users, from simple reactions or ratings to more complex error reporting or suggestion messages
- Checkout the integration guides to see all libraries, frameworks and website builder you can use with Feelback to collect user feedback